How to Use the Aerial News Stories Classification API
Use this pre-trained Aerial News Stories computer vision model to retrieve predictions with our hosted API or deploy to the edge. Learn More About Roboflow Inference
Samples from Test Set
Try this model on images
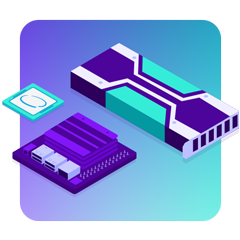
Roboflow Inference
Inference is Roboflow's open source deployment package for developer-friendly vision inference.
How to Deploy the Aerial News Stories Classification API
Using Roboflow, you can deploy your classification model to a range of environments, including:
- Luxonis OAK
- Raspberry Pi
- NVIDIA Jetson
- A Docker container
- A web page
- A Python script using the Roboflow SDK.
Below, we have instructions on how to use our deployment options.
Code Snippets
Roboflow Documentation
Look through our full documentation for more information and resources on how to utilize this model.
Example Web App
Use this model with a full fledged web application that has all sample code included.
Deploy to NVIDIA Jetson
Perform inference at the edge with a Jetson via our Docker container.
Deploy to Luxonis OAK
Perform inference at the edge with an OAK device via our Docker container.
Deploy Mobile iOS
Utilize your model on your mobile device.